C Debugging Questions Pdf
C# exercises
For my own experience as software developer, practicing exercises is an important activity to learn a programming language.
In this page you will find a lot of C# exercises to help you test your knowledge and skill of writing code in C# and practice the C# programming lessons. You will start from basic C# exercises to more complex exercises. The solution is provided for each exercise. If you have any questions regarding to each problem, you can post them at our forum. If you want to download free C# programs with source code, visit this page: Programs.
The exercises below help you practice declaring variables, getting inputs from keyboard, and outputting the results on the console window.
Introduction to operators in C and Arithmetic Operators. Relational and Logical Operators in C. Bitwise Operators in C. Operator Precedence and Associativity in C. Evaluation order of operands. Comma in C and C. Sizeof operator in C. Operands for sizeof operator. Debugging Techniques:: dbx dbx is a multi-threaded program debugger. This program is great for tracking down memory leaks. Dbx is not found on linux machines (it can be found on Solaris or other.NIX machines). Debugging Interview Questions. Difference between a crash and exception. Difference between macros and inline functions. Mfc: message maps and virtual functions. Different calling convention. Late n early binding. Garbage collector algorithm. When gc will fail to clean the memory. How to know heap size, crash dump analysis.
Exercise 1: Write C# code to declare a variable to store the age of a person. Then the output of the program is as an example shown below:
You are 20 years old.
Solution:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Csharp_exercises
{
class Program
{
static void Main(string[] args)
{
int age = 20;// declaring variable and assign 20 to it.
Console.WriteLine('You are {0} years old.',age);
Console.ReadLine();
}
C Debugging Questions With Answers Pdf
}
}
Exercise 2: Write C# code to display the asterisk pattern as shown below:
*****
*****
*****
*****
*****
Solution:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Csharp_exercises
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine('*****');
Console.WriteLine('*****');
Console.WriteLine('*****');
Console.WriteLine('*****');
Console.WriteLine('*****');
Console.ReadLine();
}
}
}
Exercise 3: Write C# code to declare two integer variables, one float variable, and one string variable and assign 10, 12.5, and 'C# programming' to them respectively. Then display their values on the screen.
Solution:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Csharp_exercises
{
class Program
{
static void Main(string[] args)
{
int x;
float y;
string s;
x = 10;
y = 12.5f;
s = 'C# programming';
Console.WriteLine(x);
Console.WriteLine(y);
Console.WriteLine(s);
Console.ReadLine();
}
}
}
Exercise 4: Write C# code to prompt a user to input his/her name and then the output will be shown as an example below:
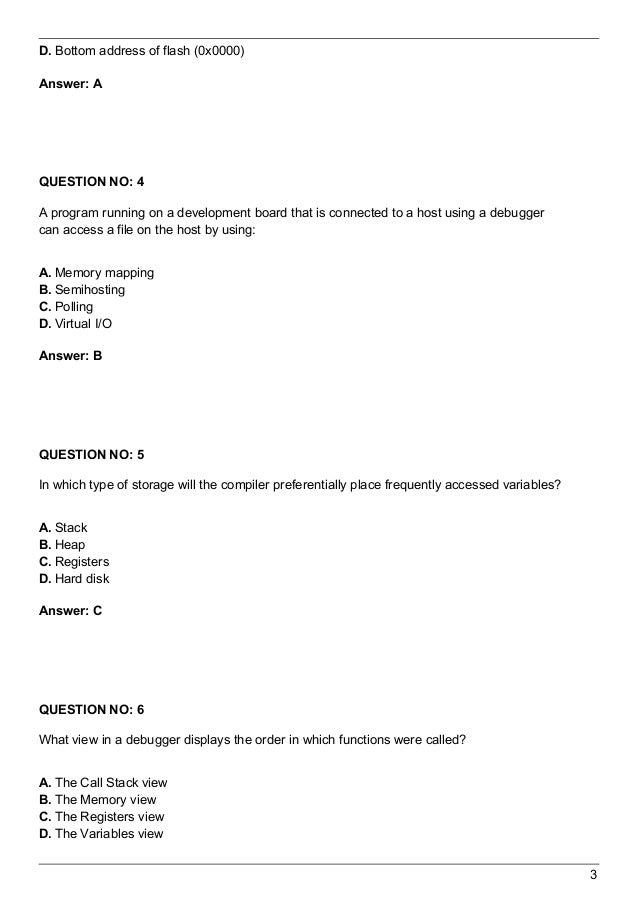
Hello John!
Solution:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Csharp_exercises
{
class Program
{
static void Main(string[] args)
{
string name;
Console.Write('Please enter your name:');
name = Console.ReadLine();
Console.WriteLine('Hello {0}!', name);
Console.ReadLine();
}
}
}
Comments
SSS improvement for Dazzler program on the 2016-03-29 2017-11-03 |
//Tiago Oliveira //Eu nao sei o q é isto nem sei se o devo utilizar 2017-06-06 |
viagra without a doctor prescr Importance The Affordable Trouble Hoax is the most superior well-being attention legislation enacted in the Collective States since the beginning of Medicare and Medicaid in 1965. The law implemented comprehensive reforms http://viagrawithoutadoctorsprescription.accountant/#26545 viagra without a doctor prescription designed to update the accessibility, affordability, and quality of condition care. 2017-02-14 |
anis fathima.s Which platform c# should run 2017-02-07 |
dharshan Generated (a,b) does not generate any na or nb n=>( where n => 1,2,3,4, . ) 2016-11-18 |
dharshan Generated (a,b) does not generate any na or nb n=>( where n => 1,2,3,4, . ) 2016-11-18 |
Nathan McCausland Cool stuff 2016-10-12 |
Grazel Diola very useful. the best. undoubtedly excellent. lovely. 2016-10-04 |
venkat hi everyone can you hepl me to write c# program to implement day for the specific date 2016-09-18 |
Alexandru @Dazzler here is a solution for your exercises, a very basic one, but a solution nevertheless: 2016-08-03 |
Nainika Really very nice blog information for this one and more technical skills are improve,i like that kind of post. 2016-05-04 |
Dazzler I am a beginner too. I have made a simple program to print multiplication table of a number. 2016-03-29 |
faraz ahmrd Develop a class Post that has following attributes 2016-03-08 |
faraz ahmrd Develop a class Post that has following attributes 2016-03-08 |
reymart fernandez hello everyone can you help me in my c# programming about function? 2016-01-13 |
jai 1.find nearest number of 89 2016-01-12 |
programmer write a program that reads in two numbers and adds them to a list if they aren't already contained in the list , when the list contains ten numbers the program displays the contents and quits 2015-12-28 |
programmer write a program that reads in two numbers and adds them to a list if they aren't already contained in the list , when the list contains ten numbers the program displays the contents and quits 2015-12-28 |
mat aleuto 2015-12-03 |
Usman Sarwar Now You may try this one 2015-11-14 |
Emmanuel Mahaso exercise 2 can also be written like so: 2015-08-11 |
Bojan I think that the solution in exercise 2 is not right. It's almost right but there is a small failure. Between every Console.WriteLine('*****'); should be a Console.WriteLine(); line to make it more accurate as the needed executable. It's minor difference but those kind of things can mean a lot in bigger programs. 2015-07-15 |
Tiotuico Daniel using System; 2015-07-09 |
Thanks ! I appericiate your effort. This web-site is useful for beginners like me. 2015-06-30 |
suresh usefull for beginers 2015-06-01 |
PAULINA NKELO this site is very useful,i m a beginner but i have already created a running program. 2015-05-19 |
Dara Anon, you are right. However, in the variables and data type exercises, i would like you to learn how to declare variables, assign values to variables, using data types, and output data on the console. It is not about using loop in this page. 2015-05-05 |
Anon I doubt the solution for 2 is the way to go. I did it with a for loop. This way you don't have however many Console.WriteLine commands for what you want, all you have to do is change the condition for the loop from 6 to 1000 if you want. Having a single command that simply repeats is way more efficient. 2015-04-28 |
Mike It would be nice to have your solution hidden. 2015-03-15 |
coolj Design a solution that prints the amount of pay a commissioned salesperson takes home 2015-02-13 |
PHORN Ya Good tutorial with the best solution for students who study in Information Technology, and I want to say many thanks 2015-02-05 |
thank you very much the tutorials were useful i thank you for posting them. 2015-01-13 |
Simon I much prefer to use - Console.WriteLine('Hello ' + Variable + '!'); Than using {0} 2014-11-16 |
Dara It refers to the first argument in the arguments list. Read this page to get more information: 2014-10-29 |
vivek In first exercise.we are declaring 2014-10-29 |
programx static void Main(string[]args{ 2014-08-16 |
Daanesh Bhathena using System; 2014-08-16 |
GURUBAKHSH YADAV This sites is helpful for programmer. 2014-08-05 |
MohitKumar Check this also for http://skillgun.com/csharp/interview-questions-and-answers 2014-08-05 |
cha tnx for this.God bless 2014-07-13 |
Ashok Gope This is this Website Tutorial is very informatic and usefull 2014-05-22 |
Jack toroni Tanks very much. 2014-03-20 |
krishna negi an u give me the GUI code with advance c#?? 2014-01-24 |
Dara This page has the explanation index_files/csharp_format_output.htm. 2014-01-12 |
sravya In first exercise.we are declaring 2014-01-04 |
Dara You will use a loop and Substring function to generate the output. Try the following code: 2013-11-20 |
vidhya prg output is 2013-11-20 |
Ather rar Tutorial on this website is very informatic and usefull for selflearning. much much appreciate for this efforts 2013-10-13 |